- Published on
Manage component data dynamically with React State
- Authors
- Name
- Kunwar Pratap
- @Kunwar_P_15
- 388 words2 min read
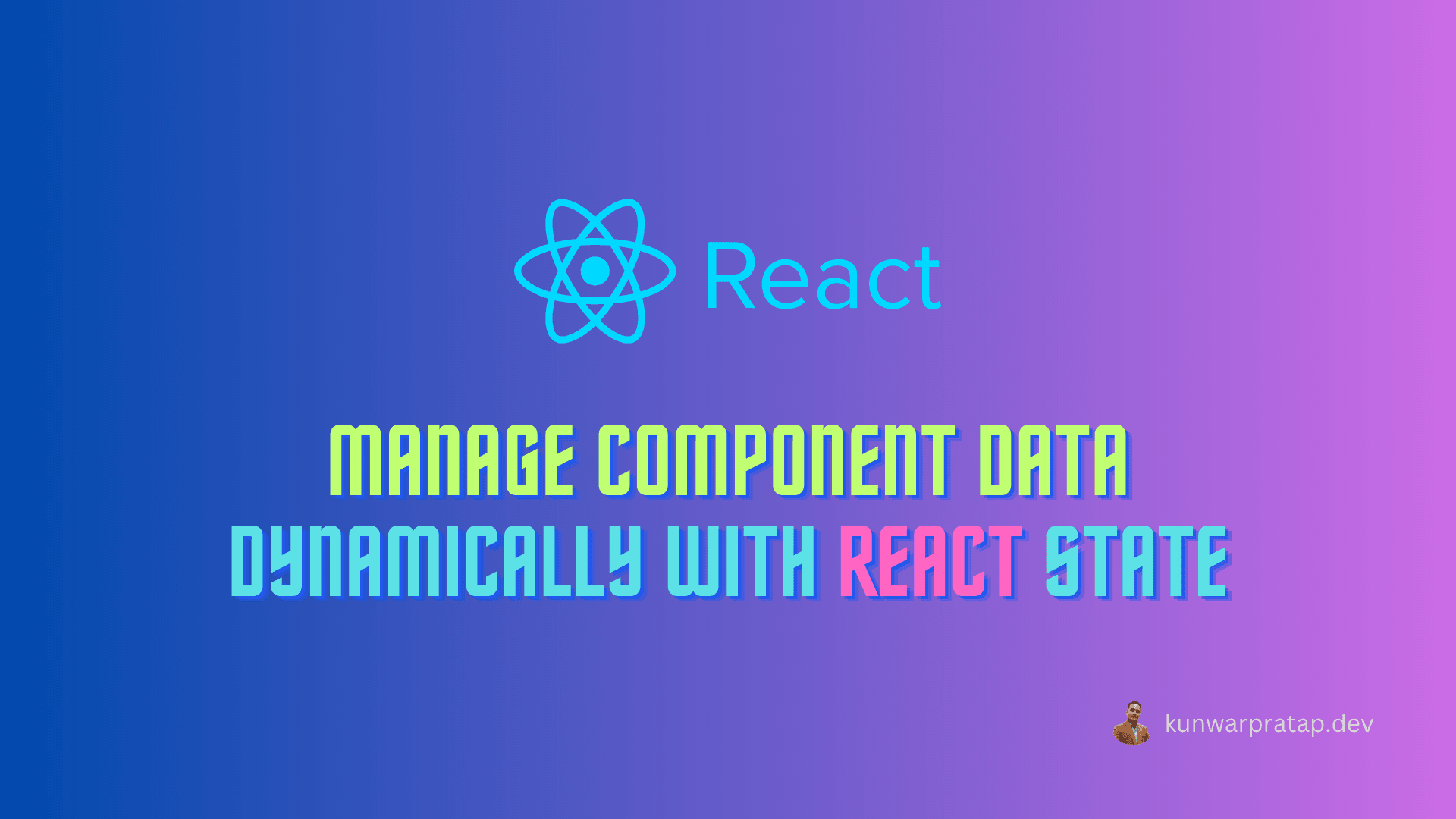
Introduction
In React, a state is an object of a component that holds values. These values can represent various aspects of the component's behavior or appearance, such as whether a button is clicked, the text entered into a form field, or which page is viewed. State allows React components to manage and keep track of their own data, making it possible to create dynamic and interactive user interfaces. When the state of a component changes, React automatically re-renders the component for reflecting the updated state.
In summary, state in React components is used to manage and represent the current state or condition of the component, which can include various types of data or values.
For example:
We are building a blog post editor component in React. The component allows users to create new blog posts by entering a title and description.
Here's how can we use state for achieve this:
import React, { useState } from 'react'
function BlogPostEditor() {
// state to hold the blog post data
const [blogPost, setBlogPost] = useState({
title: '',
description: '',
})
// Function to handle the changes in input fields
const handleChange = (e) => {
const { name, value } = e.target
setBlogPost({ ...blogPost, [name]: value })
}
// Function to handle the submission of the blog post
const handleSubmit = (e) => {
e.preventDefault()
// Perform actions according to need like saving the blog post to a database
console.log('Blog post Submitted:', blogPost)
// Reset the form fields after submission of form
setBlogPost({ title: '', description: '' })
}
return (
<div>
<h2>Create a New Blog Post</h2>
<form onSubmit={handleSubmit}>
<div>
<input type="text" name="title" value={blogPost.title} onChange={handleChange} />
</div>
<div>
<textarea name="description" value={blogPost.description} onChange={handleChange} />
</div>
<button type="submit">Submit</button>
</form>
</div>
)
}
export default BlogPostEditor
In this example:
We use the useState hook from React to define a piece of state called blogPost, which represents the data of the blog post being created.
The handleChange function updates the blogPost state as the user types in the input fields for title and description.
The handleSubmit function is called when the user submits the form.
The component renders input fields for the title and description of the blog post.
When the form is submitted, the form fields are reset to their initial state.